Why Do We Need Flows in Android Development?
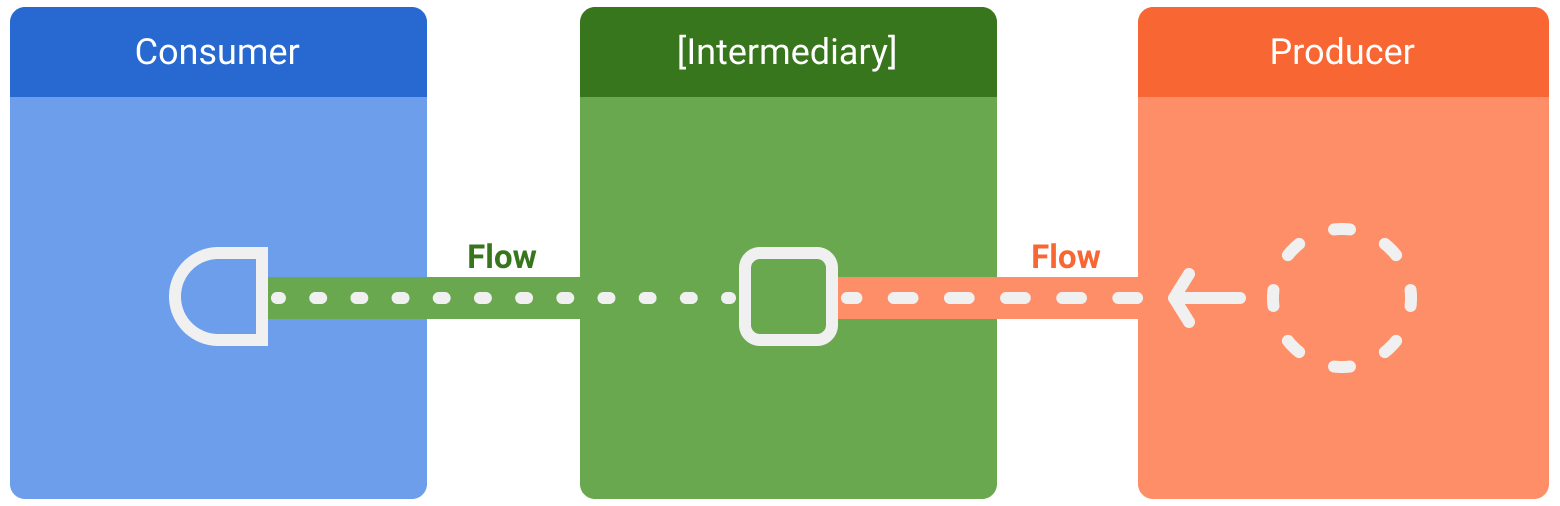
In modern Android development, building apps efficiently and maintaining high performance are paramount. One key tool that developers use to manage asynchronous programming and handle dynamic data streams is Kotlin’s Flows. Introduced as part of the Kotlin Coroutines API, Flows help streamline how we handle reactive programming in Android. But why are Flows so important in Android development? Let’s dive deep into the reasons behind their growing popularity, their usage, and how they significantly enhance development.
What Are Flows in Kotlin?
Before exploring their importance, it’s essential to understand what Flows are. In simple terms, Flows are a reactive stream that emit a sequence of values asynchronously. Flows are part of Kotlin’s Coroutines API, which helps us manage and simplify asynchronous operations. They can be thought of as Cold Streams, meaning they only start emitting data when there is an active subscriber or collector.
Unlike older reactive frameworks such as RxJava, Flows provide a cleaner, more readable API with built-in coroutines support, making them more efficient for handling streams of data in Android applications.
Why Are Flows Important in Android Development?
1. Handling Asynchronous Data Efficiently
In Android development, most operations that interact with external systems (like networking, database access, or file handling) are asynchronous by nature. Using Kotlin’s Flow API allows developers to handle these tasks in a more structured and manageable way. Instead of manually managing multiple threads or callbacks, we can utilize Flows to make our code cleaner and easier to read.
2. Streamlining Data Streams
Data streams are common in Android applications, especially when dealing with continuous updates, such as location tracking, sensor data, or live updates from a server. With Flows, we can seamlessly handle streams of data without worrying about state management or memory leaks. This not only reduces the potential for bugs but also makes the development process faster and more straightforward.
3. Replacing Callbacks with a Declarative Approach
Traditional Android development often relies on callback-based systems, especially for long-running operations like API calls. Callbacks can quickly become nested, hard to debug, and difficult to maintain. Flows replace the need for callback-heavy logic by providing a declarative way to handle asynchronous data.
With a Flow, we simply declare what needs to be done when the data arrives, rather than how to orchestrate the entire process. This makes the code much easier to understand and maintain, especially in complex Android apps.
4. Backpressure Handling
When dealing with large streams of data or data coming at unpredictable rates (for example, when receiving push notifications from a server), handling backpressure becomes essential. Flows are designed to handle backpressure gracefully, ensuring that your application remains responsive, regardless of how much data is being emitted. This is a significant improvement over older systems like RxJava, where manual backpressure handling can introduce complexities and potential errors.
5. Integration with Jetpack Components
One of the key reasons to use Flows in Android development is their seamless integration with Jetpack components. For instance, we can use Flows with ViewModels, LiveData, Room database, and other Android libraries to create efficient, lifecycle-aware components.
Flows also make it easier to tie asynchronous tasks into the lifecycle of Android components like activities or fragments, avoiding common pitfalls like memory leaks or crashes due to improper lifecycle management.
How Do Flows Work in Android Development?
1. Flow Creation
Creating a Flow is straightforward. We can use flow builders like flow {}
to emit values. Here’s a simple example:
1
2
3
4
5
6
val dataFlow = flow {
for (i in 1..5) {
emit(i)
delay(1000L) // Simulate some asynchronous work
}
}
In this example, we are emitting values from 1 to 5 with a delay of one second between each emission.
2. Collecting Data from a Flow
The Flow does not execute until we collect its values. Here’s how we collect values from the Flow:
1
2
3
dataFlow.collect { value ->
println("Received value: $value")
}
The collector only runs when there is active data being emitted by the Flow, making it highly efficient.
3. Combining Flows
Another powerful feature of Flows is their ability to combine multiple data streams. If we have multiple data sources that we want to combine, Flows make it easy:
1
2
3
4
5
6
7
8
val flow1 = flowOf(1, 2, 3)
val flow2 = flowOf("A", "B", "C")
flow1.zip(flow2) { num, letter ->
"$num$letter"
}.collect { combined ->
println(combined) // Outputs: 1A, 2B, 3C
}
This combination feature is crucial for scenarios like synchronizing UI updates based on multiple asynchronous sources of data.
Common Use Cases for Flows in Android Development
1. Database Operations with Room
One of the most common use cases of Flows in Android is working with the Room persistence library. The Room library supports Flow as a return type for database queries, allowing real-time updates to your UI whenever the underlying database changes:
1
2
3
4
5
@Dao
interface UserDao {
@Query("SELECT * FROM users")
fun getAllUsers(): Flow<List<User>>
}
This ensures that any changes to the users
table will automatically trigger updates to the UI without any additional code.
2. Real-time API Data Fetching
Another popular use of Flows is in fetching data from APIs that provide real-time updates. For instance, using Flows with a WebSocket or polling API allows us to handle updates efficiently:
1
2
3
4
5
6
7
val apiFlow = flow {
while (true) {
val response = apiService.getLatestData()
emit(response)
delay(5000L) // Polling every 5 seconds
}
}
3. Managing UI State
Flows can be effectively used for managing and updating UI state. Since they emit a continuous stream of data, they are perfect for scenarios where the UI needs to be updated frequently based on incoming data (e.g., in live sports score apps, financial tracking apps, etc.).
Best Practices for Using Flows in Android Development
-
Use Operators: Flows come with a variety of powerful operators like
map
,filter
,flatMapConcat
, andzip
, which allow us to transform, combine, and manipulate data streams. -
Lifecycle Awareness: When using Flows with Android components, it’s crucial to ensure they are lifecycle-aware. Use viewModelScope or lifecycleScope to automatically cancel Flows when a ViewModel or UI component is destroyed.
-
Error Handling: Always ensure proper error handling with operators like
catch {}
. This helps in managing unexpected errors gracefully. -
Cold Flows vs Hot Flows: Understand the difference between cold and hot flows. Cold Flows, like those created with
flow {}
, start emitting data only when they are collected. On the other hand, StateFlow and SharedFlow are hot flows, which emit data even without an active collector.
Conclusion
Kotlin’s Flow API provides Android developers with a powerful tool for managing asynchronous data streams, offering improved readability, efficiency, and performance over traditional approaches. By utilizing Flows, we can create cleaner, more maintainable code that responds seamlessly to dynamic data changes, integrates smoothly with Android’s lifecycle components, and simplifies asynchronous operations.
For more information about how to boost traffic on your website, visit –> The Insider’s Views.